This is a bit of a niche blog post, because you don’t actually need this to make your Azure Function work :). When you create a new HTTP-triggered Azure Function (using C# code) in Visual Studio, you’ll get the following boilerplate code:
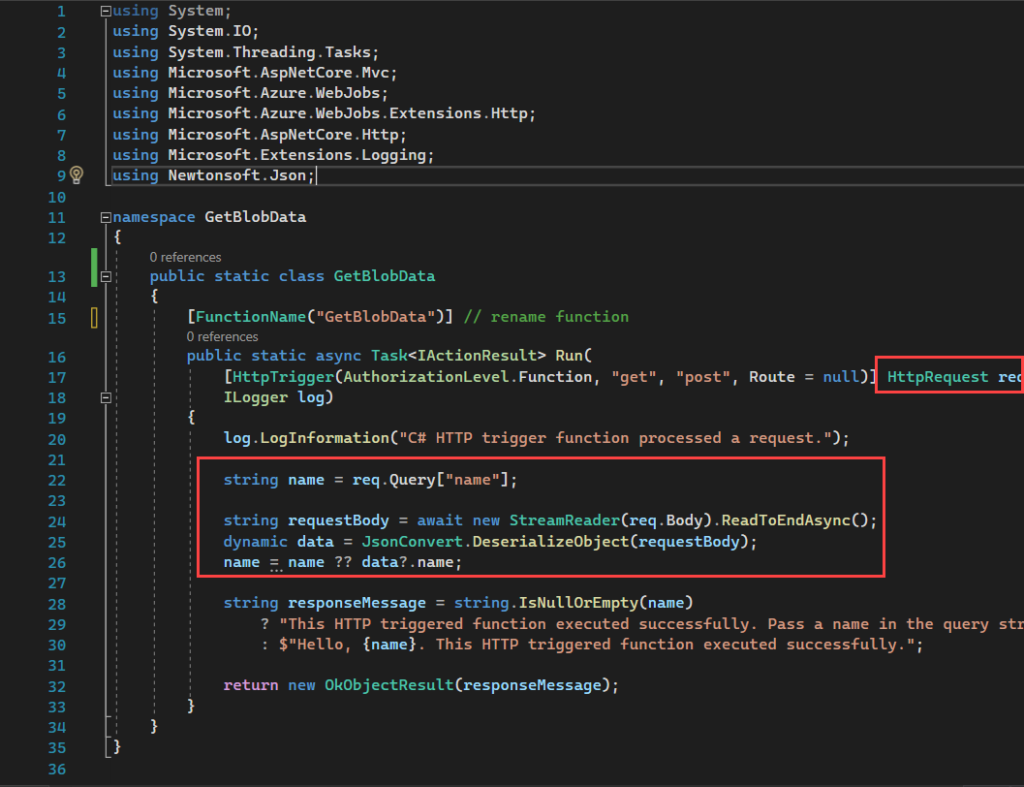
This code reads the body of the HTTP request (called req) and tries to extract the property name. Once directly, once through deserializing the JSON of the body. This means that for every function execution, the code has to parse the body of the request and find all the parameters, not knowing what to expect.
However, when you design your function, you probably know upfront how the body is going to look like. This begs the question: is there a way to describe the JSON structure of the body? Turns it out you can (otherwise this blog post wouldn’t have a point). And it just takes a couple of lines of code.
Let’s start by adding a new class to our project (I’ve put it in a folder called Model, as recommended by a colleague who is better at C# than I am) and call it BodyRequest.
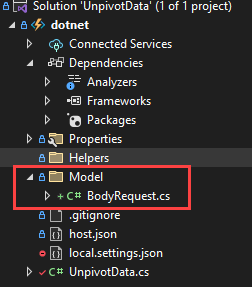
In this class, we specify a constructor for each field of the body. Suppose we want to provide the function with the following body:
{ "container":"my-datalake", "folder":"clean/excel-files/", "filename":"myExcelFile.xlsx", "tablename":"mySQLTable" }
This would result in the following class definition:
namespace dotnet.Model // dotnet is the name of my project in Visual Studio { public class BodyRequest { public string container { get; set; } public string folder { get; set; } public string filename { get; set; } public string tablename { get; set; } } }
In the Azure Function itself, replace the HttpRequest with an instance of this new class:
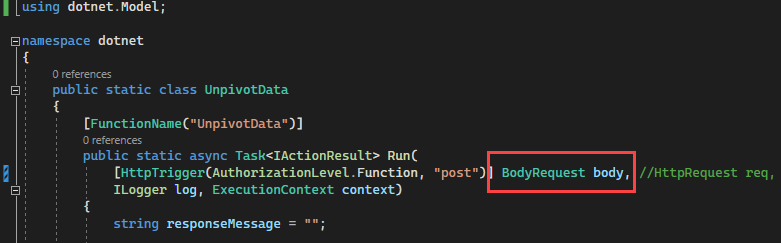
And now we can just read the properties of this class in the function without any deserialization:
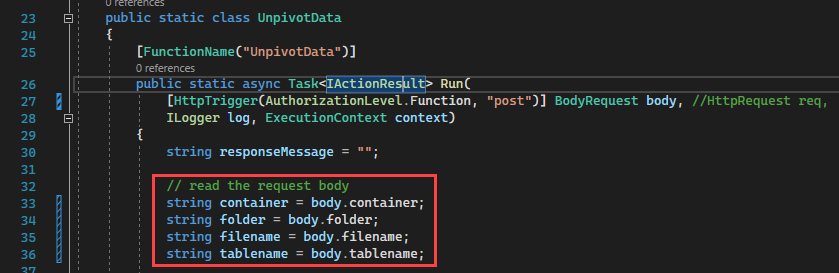
What happens if a field is missing from the body? For example:
{ "container":"my-datalake", "folder":"clean/excel-files/", "tablename":"mySQLTable" }
In this case, the filename will be set to null. If the body contains a field that isn’t specified in the format of the body, it will be ignored. For example:
{ "container":"my-datalake", "folder":"clean/excel-files/", "test":123 }
You’ll probably get an error when you specify a value with a data type which cannot be implicitly converted, for example string to int. And that’s it. More cleaner (and a tiny bit more efficient) code.
The post How to Specify the Format of the Request Body of an Azure Function first appeared on Under the kover of business intelligence.